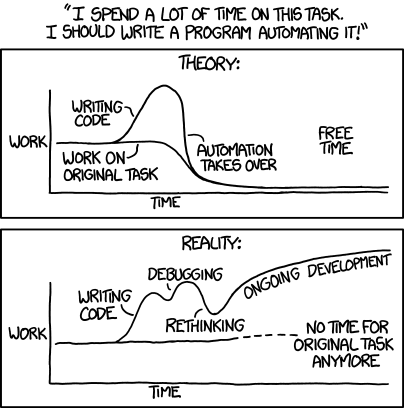
Lets face it we are all a little bit lazy and why do something the hard way when you can get a computer to do it for you. This topic is to share tasks you’ve automated to make your life easier or more efficient, be it in Bash, Python, C, PowerShell, Excel, hell even Redstone, if you’ve automated it I want to see it.
mp3 conversion
I made this script a while ago to convert my music collection to mp3. It takes three arguments either wav, flac, or ogg and converts all files in the current directory to mp3.
#!/bin/bash
# loop through files in the currently active directory
for f in *.$1
do
# case statement for arguments (you can convert wav, flac, and ogg to mp3)
case $1 in
wav)
# converts wav files to mp3 using lame
lame -b320 "$f" "${f%.*}".mp3
;;
flac)
# converts flac files to mp3 using flac/lame
flac -cd "$f" | lame -b320 - "${f%.*}".mp3
;;
ogg)
# converts ogg to mp3 using oggdec/lame
oggdec "$f" -o - | lame -b320 - "${f%.*}".mp3
;;
*)
echo "Usage: $0 {wav|flac|ogg}"
esac
shopt -s nullglob
# check for mp3 files in current directory
if [[ -n $(echo *.mp3) ]]; then
# check if mp3 directory exists
if [ ! -d "mp3" ]; then
# creates mp3 directory if it doesn't already exist
mkdir mp3
fi
# move all mp3 files to the mp3 directory
mv *.mp3 mp3/
fi
done
FLAC conversion
After a while of using mp3 for my music I decided to switch to FLAC so adapted the script above to convert wav files to flac:
#!/bin/bash
# loop through files in currently active directory
for f in *.wav
do
# converts wav files to flac using sox
sox "$f" "${f%.*}".flac
shopt -s nullglob
#check for flac files in current directory
if [[ -n $(echo *.flac) ]]; then
# check if flac directory exists
if [ ! -d "flac" ]; then
# creates flac direcotry if it doesn't already exist
mkdir flac
fi
# move all flac files to the flac directory
mv *.flac flac/
fi
done
LFS Chroot
When I built Linux from Stretch I often needed to detach my usb flash drive to test it but also center the chroot again later so I made this. it takes two arguments m and u, the first mounts everything and enters the chroot and the second unmounts and cleans everything up.
#!/bin/bash
export LFS=/mnt/lfs
case $1 in
m)
mount -v -t ext4 /dev/sde1 $LFS
mount -v -t ext4 /dev/sde2 $LFS/home
mount -v --bind /dev $LFS/dev
mount -vt devpts devpts $LFS/dev/pts -o gid=5,mode=620
mount -vt proc proc $LFS/proc
mount -vt sysfs sysfs $LFS/sys
mount -vt tmpfs tmpfs $LFS/run
if [ -h $LFS/dev/shm ]; then
mkdir -pv $LFS/$(readlink $LFS/dev/shm)
fi
chroot "$LFS" /usr/bin/env -i \
HOME=/root TERM="$TERM" PS1='\u:\w\$ ' \
PATH=/bin:/usr/bin:/sbin:/usr/sbin \
/bin/bash --login
;;
u)
umount -v $LFS/dev/pts
umount -v $LFS/dev
umount -v $LFS/run
umount -v $LFS/proc
umount -v $LFS/sys
umount -v $LFS/home
umount -v $LFS
;;
*)
echo "Usage: $0 {m|u}"
esac
I also made a script to automate the creation of a bootable Windows flash drive which I posted in more detail here:
Thats it for now, I’ll post new stuff later when I have more to show.